Write The Shortest Program To Check If A Binary Tree Is BalancedConvert binary search tree into ordered linked listPrint a non-clashing binary search treeTree traversingPre-order + post-order to in-orderIs it a Linearized Tree? (Breadth-first Edition)Compute the height of a radix treeRooting for Trees With the Right NodesBinary tree rotationsIs this a BST pre-order traversal?Write The Shortest Program to Calculate Height of a Binary Tree
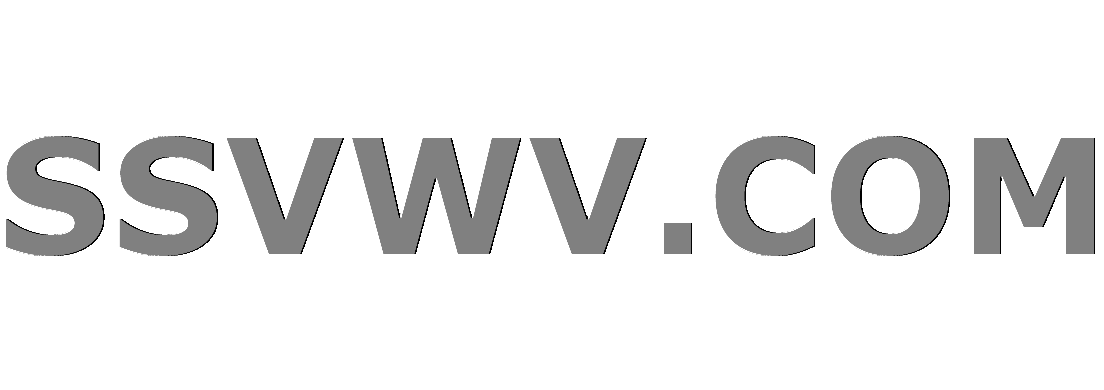
Multi tool use
Why do dragons like shiny stuff?
Conditional probability of dependent random variables
How to win against ants
How do I show and not tell a backstory?
ZFS on Linux: Which mountpoint option when mounting manually per script?
Has J.J.Jameson ever found out that Peter Parker is Spider-Man?
Did Logical Positivism fail because it simply denied human emotion?
Awk to get all my regular users in shadow
Why do rocket engines use nitrogen actuators to operate the fuel/oxidiser valves instead of electric servos?
Piece de Resistance - Introduction & Ace and A's
What printing process is this?
If someone else uploads my GPL'd code to Github without my permission, is that a copyright violation?
Are the related objects in an SOQL query shared?
Is an "are" omitted in this sentence
A verb for when some rights are not violated?
Can attackers change the public key of certificate during the SSL handshake
What do "unsettled funds" mean?
How to call made-up data?
How to design an effective polearm-bow hybrid?
C# TCP server/client class
Why did the US Airways Flight 1549 passengers stay on the wings?
Generate random number in Unity without class ambiguity
Getting Lost in the Caves of Chaos
Why are there yellow dot stickers on the front doors of businesses in Russia?
Write The Shortest Program To Check If A Binary Tree Is Balanced
Convert binary search tree into ordered linked listPrint a non-clashing binary search treeTree traversingPre-order + post-order to in-orderIs it a Linearized Tree? (Breadth-first Edition)Compute the height of a radix treeRooting for Trees With the Right NodesBinary tree rotationsIs this a BST pre-order traversal?Write The Shortest Program to Calculate Height of a Binary Tree
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
For each node in a balanced, binary tree, the maximum difference in the heights of the left child subtree and the right child subtree are at most 1.
The height of a binary tree is the distance from the root node to the node child that is farthest from the root.
Below is an example:
2 <-- root: Height 1
/
7 5 <-- Height 2
/
2 6 9 <-- Height 3
/ /
5 11 4 <-- Height 4
Height of binary tree: 4
The following are binary trees and a report on whether or not they are balanced:
The tree above is unbalanced.
The above tree is balanced.
Write the shortest program possible that accepts as input the root of a binary tree and returns a falsey value if the tree is unbalanced and a truthy value if the tree is balanced.
Input
The root of a binary tree. This may be in the form of a reference to the root object or even a list that is a valid representation of a binary tree.
Output
Returns truthy value: If the tree is balanced
Returns falsey value: If the tree is unbalanced.
Definition of a Binary Tree
A tree is an object that contains a value and either two other trees or pointers to them.
The structure of the binary tree looks something like the following:
typedef struct T
struct T *l;
struct T *r;
int v;
T;
If using a list representation for a binary tree, it may look something like the following:
[root_value, left_node, right_node]
code-golf binary-tree tree-traversal
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
For each node in a balanced, binary tree, the maximum difference in the heights of the left child subtree and the right child subtree are at most 1.
The height of a binary tree is the distance from the root node to the node child that is farthest from the root.
Below is an example:
2 <-- root: Height 1
/
7 5 <-- Height 2
/
2 6 9 <-- Height 3
/ /
5 11 4 <-- Height 4
Height of binary tree: 4
The following are binary trees and a report on whether or not they are balanced:
The tree above is unbalanced.
The above tree is balanced.
Write the shortest program possible that accepts as input the root of a binary tree and returns a falsey value if the tree is unbalanced and a truthy value if the tree is balanced.
Input
The root of a binary tree. This may be in the form of a reference to the root object or even a list that is a valid representation of a binary tree.
Output
Returns truthy value: If the tree is balanced
Returns falsey value: If the tree is unbalanced.
Definition of a Binary Tree
A tree is an object that contains a value and either two other trees or pointers to them.
The structure of the binary tree looks something like the following:
typedef struct T
struct T *l;
struct T *r;
int v;
T;
If using a list representation for a binary tree, it may look something like the following:
[root_value, left_node, right_node]
code-golf binary-tree tree-traversal
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
For each node in a balanced, binary tree, the maximum difference in the heights of the left child subtree and the right child subtree are at most 1.
The height of a binary tree is the distance from the root node to the node child that is farthest from the root.
Below is an example:
2 <-- root: Height 1
/
7 5 <-- Height 2
/
2 6 9 <-- Height 3
/ /
5 11 4 <-- Height 4
Height of binary tree: 4
The following are binary trees and a report on whether or not they are balanced:
The tree above is unbalanced.
The above tree is balanced.
Write the shortest program possible that accepts as input the root of a binary tree and returns a falsey value if the tree is unbalanced and a truthy value if the tree is balanced.
Input
The root of a binary tree. This may be in the form of a reference to the root object or even a list that is a valid representation of a binary tree.
Output
Returns truthy value: If the tree is balanced
Returns falsey value: If the tree is unbalanced.
Definition of a Binary Tree
A tree is an object that contains a value and either two other trees or pointers to them.
The structure of the binary tree looks something like the following:
typedef struct T
struct T *l;
struct T *r;
int v;
T;
If using a list representation for a binary tree, it may look something like the following:
[root_value, left_node, right_node]
code-golf binary-tree tree-traversal
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
For each node in a balanced, binary tree, the maximum difference in the heights of the left child subtree and the right child subtree are at most 1.
The height of a binary tree is the distance from the root node to the node child that is farthest from the root.
Below is an example:
2 <-- root: Height 1
/
7 5 <-- Height 2
/
2 6 9 <-- Height 3
/ /
5 11 4 <-- Height 4
Height of binary tree: 4
The following are binary trees and a report on whether or not they are balanced:
The tree above is unbalanced.
The above tree is balanced.
Write the shortest program possible that accepts as input the root of a binary tree and returns a falsey value if the tree is unbalanced and a truthy value if the tree is balanced.
Input
The root of a binary tree. This may be in the form of a reference to the root object or even a list that is a valid representation of a binary tree.
Output
Returns truthy value: If the tree is balanced
Returns falsey value: If the tree is unbalanced.
Definition of a Binary Tree
A tree is an object that contains a value and either two other trees or pointers to them.
The structure of the binary tree looks something like the following:
typedef struct T
struct T *l;
struct T *r;
int v;
T;
If using a list representation for a binary tree, it may look something like the following:
[root_value, left_node, right_node]
code-golf binary-tree tree-traversal
code-golf binary-tree tree-traversal
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 8 hours ago
T. SalimT. Salim
1401 silver badge9 bronze badges
1401 silver badge9 bronze badges
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
T. Salim is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
$begingroup$
Jelly, 11 bytes
ḊµŒḊ€IỊ;߀Ạ
Try it online!
The empty tree is represented by []
.
$endgroup$
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
add a comment |
$begingroup$
Prolog (SWI), 49 bytes
N+_/B/C:-X+B,Y+C,abs(X-Y)<2,N is max(X,Y)+1.
0+e.
Try it online!
Represents trees as Value/Left_Child/Right_Child
, with the empty tree being the atom e
. Defines +/2
, which outputs through success or failure, with an unbound variable (or one already equal to the tree's height) on the left and the tree on the right--if the height argument is unacceptable, add 9 bytes to define -T:-_+T.
.
N + _/B/C :- % If the second argument is a tree of the form _Value/B/C,
X+B, % X is the height of its left child which is balanced,
Y+C, % Y is the height of its right child which is balanced,
abs(X-Y) < 2, % the absolute difference between X and Y is strictly less than 2,
N is max(X,Y)+1. % and N is the height of the full tree.
0 + e. % If, on the other hand, the second argument is e, the first is 0.
$endgroup$
add a comment |
$begingroup$
JavaScript, 162 bytes
f=x=>for(f=0,s=[[x,1]];s[0];)if(!((d=(t=s.pop())[0]).a&&d.breturn 1
Try it online!
The format of the input is an object
root=a:node,b:node,c:value
Explanation
for(f=0,s=[[x,1]];s[0];)
Continuing the breadth first search, return zero if any element is two deeper than the depth of the first node missing branches.
return 1}
If no such node is found, return 1
$endgroup$
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "200"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
T. Salim is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f189285%2fwrite-the-shortest-program-to-check-if-a-binary-tree-is-balanced%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
Jelly, 11 bytes
ḊµŒḊ€IỊ;߀Ạ
Try it online!
The empty tree is represented by []
.
$endgroup$
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
add a comment |
$begingroup$
Jelly, 11 bytes
ḊµŒḊ€IỊ;߀Ạ
Try it online!
The empty tree is represented by []
.
$endgroup$
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
add a comment |
$begingroup$
Jelly, 11 bytes
ḊµŒḊ€IỊ;߀Ạ
Try it online!
The empty tree is represented by []
.
$endgroup$
Jelly, 11 bytes
ḊµŒḊ€IỊ;߀Ạ
Try it online!
The empty tree is represented by []
.
answered 8 hours ago


Erik the OutgolferErik the Outgolfer
35.2k4 gold badges30 silver badges110 bronze badges
35.2k4 gold badges30 silver badges110 bronze badges
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
add a comment |
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
$begingroup$
Thanks Erik for being amongst the first to answer this question. Jelly certainly is a very popular language on this site. I think I should take the liberty to implement this language. Good to learn from a robust golf-scripting language.
$endgroup$
– T. Salim
6 hours ago
add a comment |
$begingroup$
Prolog (SWI), 49 bytes
N+_/B/C:-X+B,Y+C,abs(X-Y)<2,N is max(X,Y)+1.
0+e.
Try it online!
Represents trees as Value/Left_Child/Right_Child
, with the empty tree being the atom e
. Defines +/2
, which outputs through success or failure, with an unbound variable (or one already equal to the tree's height) on the left and the tree on the right--if the height argument is unacceptable, add 9 bytes to define -T:-_+T.
.
N + _/B/C :- % If the second argument is a tree of the form _Value/B/C,
X+B, % X is the height of its left child which is balanced,
Y+C, % Y is the height of its right child which is balanced,
abs(X-Y) < 2, % the absolute difference between X and Y is strictly less than 2,
N is max(X,Y)+1. % and N is the height of the full tree.
0 + e. % If, on the other hand, the second argument is e, the first is 0.
$endgroup$
add a comment |
$begingroup$
Prolog (SWI), 49 bytes
N+_/B/C:-X+B,Y+C,abs(X-Y)<2,N is max(X,Y)+1.
0+e.
Try it online!
Represents trees as Value/Left_Child/Right_Child
, with the empty tree being the atom e
. Defines +/2
, which outputs through success or failure, with an unbound variable (or one already equal to the tree's height) on the left and the tree on the right--if the height argument is unacceptable, add 9 bytes to define -T:-_+T.
.
N + _/B/C :- % If the second argument is a tree of the form _Value/B/C,
X+B, % X is the height of its left child which is balanced,
Y+C, % Y is the height of its right child which is balanced,
abs(X-Y) < 2, % the absolute difference between X and Y is strictly less than 2,
N is max(X,Y)+1. % and N is the height of the full tree.
0 + e. % If, on the other hand, the second argument is e, the first is 0.
$endgroup$
add a comment |
$begingroup$
Prolog (SWI), 49 bytes
N+_/B/C:-X+B,Y+C,abs(X-Y)<2,N is max(X,Y)+1.
0+e.
Try it online!
Represents trees as Value/Left_Child/Right_Child
, with the empty tree being the atom e
. Defines +/2
, which outputs through success or failure, with an unbound variable (or one already equal to the tree's height) on the left and the tree on the right--if the height argument is unacceptable, add 9 bytes to define -T:-_+T.
.
N + _/B/C :- % If the second argument is a tree of the form _Value/B/C,
X+B, % X is the height of its left child which is balanced,
Y+C, % Y is the height of its right child which is balanced,
abs(X-Y) < 2, % the absolute difference between X and Y is strictly less than 2,
N is max(X,Y)+1. % and N is the height of the full tree.
0 + e. % If, on the other hand, the second argument is e, the first is 0.
$endgroup$
Prolog (SWI), 49 bytes
N+_/B/C:-X+B,Y+C,abs(X-Y)<2,N is max(X,Y)+1.
0+e.
Try it online!
Represents trees as Value/Left_Child/Right_Child
, with the empty tree being the atom e
. Defines +/2
, which outputs through success or failure, with an unbound variable (or one already equal to the tree's height) on the left and the tree on the right--if the height argument is unacceptable, add 9 bytes to define -T:-_+T.
.
N + _/B/C :- % If the second argument is a tree of the form _Value/B/C,
X+B, % X is the height of its left child which is balanced,
Y+C, % Y is the height of its right child which is balanced,
abs(X-Y) < 2, % the absolute difference between X and Y is strictly less than 2,
N is max(X,Y)+1. % and N is the height of the full tree.
0 + e. % If, on the other hand, the second argument is e, the first is 0.
answered 7 hours ago


Unrelated StringUnrelated String
3,1252 gold badges3 silver badges16 bronze badges
3,1252 gold badges3 silver badges16 bronze badges
add a comment |
add a comment |
$begingroup$
JavaScript, 162 bytes
f=x=>for(f=0,s=[[x,1]];s[0];)if(!((d=(t=s.pop())[0]).a&&d.breturn 1
Try it online!
The format of the input is an object
root=a:node,b:node,c:value
Explanation
for(f=0,s=[[x,1]];s[0];)
Continuing the breadth first search, return zero if any element is two deeper than the depth of the first node missing branches.
return 1}
If no such node is found, return 1
$endgroup$
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
add a comment |
$begingroup$
JavaScript, 162 bytes
f=x=>for(f=0,s=[[x,1]];s[0];)if(!((d=(t=s.pop())[0]).a&&d.breturn 1
Try it online!
The format of the input is an object
root=a:node,b:node,c:value
Explanation
for(f=0,s=[[x,1]];s[0];)
Continuing the breadth first search, return zero if any element is two deeper than the depth of the first node missing branches.
return 1}
If no such node is found, return 1
$endgroup$
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
add a comment |
$begingroup$
JavaScript, 162 bytes
f=x=>for(f=0,s=[[x,1]];s[0];)if(!((d=(t=s.pop())[0]).a&&d.breturn 1
Try it online!
The format of the input is an object
root=a:node,b:node,c:value
Explanation
for(f=0,s=[[x,1]];s[0];)
Continuing the breadth first search, return zero if any element is two deeper than the depth of the first node missing branches.
return 1}
If no such node is found, return 1
$endgroup$
JavaScript, 162 bytes
f=x=>for(f=0,s=[[x,1]];s[0];)if(!((d=(t=s.pop())[0]).a&&d.breturn 1
Try it online!
The format of the input is an object
root=a:node,b:node,c:value
Explanation
for(f=0,s=[[x,1]];s[0];)
Continuing the breadth first search, return zero if any element is two deeper than the depth of the first node missing branches.
return 1}
If no such node is found, return 1
edited 5 hours ago
answered 6 hours ago


fəˈnɛtɪkfəˈnɛtɪk
3,7362 gold badges6 silver badges37 bronze badges
3,7362 gold badges6 silver badges37 bronze badges
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
add a comment |
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
1
1
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
$begingroup$
There is probably some way to do the breadth first search better but I couldn't think of it.
$endgroup$
– fəˈnɛtɪk
5 hours ago
add a comment |
T. Salim is a new contributor. Be nice, and check out our Code of Conduct.
T. Salim is a new contributor. Be nice, and check out our Code of Conduct.
T. Salim is a new contributor. Be nice, and check out our Code of Conduct.
T. Salim is a new contributor. Be nice, and check out our Code of Conduct.
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f189285%2fwrite-the-shortest-program-to-check-if-a-binary-tree-is-balanced%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DEafNI,Ajx047,KXlVH4,3p O hdWwL8zYHkrYGiwjcwrcji5yY,SfT5W18BCMc2Fub,U0