Mutable named tuple with default value and conditional rounding supportConditional statements with doctor and patient informationTree structure with support for inorder and preorder traversalFunctions with mutable and non-mutable named tuplesLoop an array of dictionary with keys containg sets; comparing each key, value pair; and combining dictionariesDynamic class instancing (with conditional parameters and methods) based on a dictionarySimplify definition of a dictionary with default return valueMutable Named Tuple - or - Slotted Data Structure
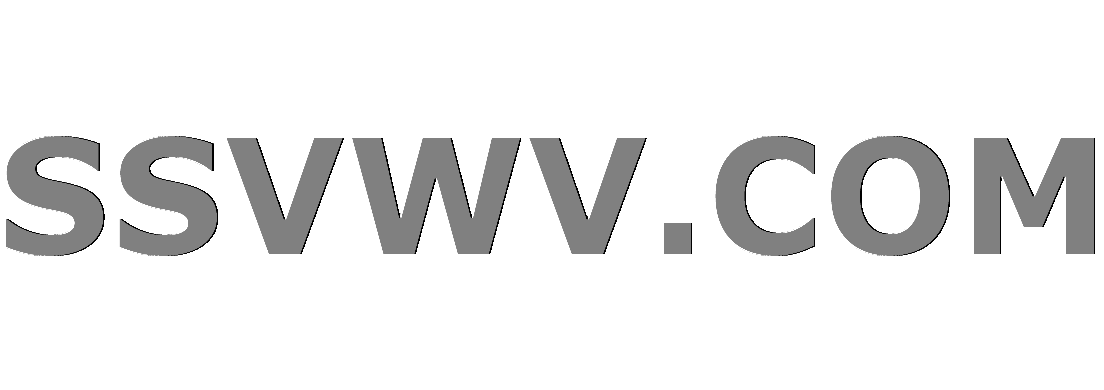
Multi tool use
Parallel resistance in electric circuits
Stucturing information on this trade show banner
Difference in using Lightning Component <lighting:badge/> and Normal DOM with slds <span class="slds-badge"></span>? Which is Better and Why?
Should you only use colons and periods in dialogues?
What officially disallows US presidents from driving?
Does deswegen have another meaning than "that is why"?
Make 2019 with single digits
Where to disclose a zero day vulnerability
In Germany, how can I maximize the impact of my charitable donations?
What hard drive connector is this?
How do I say "quirky" in German without sounding derogatory?
Make 1998 using the least possible digits 8
What was the motivation for the invention of electric pianos?
POSIX compatible way to get user name associated with a user ID
What exactly is a marshrutka (маршрутка)?
Can I fix my boots by gluing the soles back on?
Bash, import output from command as command
Finding the number of digits of a given integer.
Why don't Wizards use wrist straps to protect against disarming charms?
Is "you will become a subject matter expert" code for "you'll be working on your own 100% of the time"?
Were Roman public roads build by private companies?
Why did they ever make smaller than full-frame sensors?
What is and what isn't ullage in rocket science?
Can I toggle Do Not Disturb on/off on my Mac as easily as I can on my iPhone?
Mutable named tuple with default value and conditional rounding support
Conditional statements with doctor and patient informationTree structure with support for inorder and preorder traversalFunctions with mutable and non-mutable named tuplesLoop an array of dictionary with keys containg sets; comparing each key, value pair; and combining dictionariesDynamic class instancing (with conditional parameters and methods) based on a dictionarySimplify definition of a dictionary with default return valueMutable Named Tuple - or - Slotted Data Structure
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
I have following code to create a mutable namedtuple. based my understand I can use dataclass to do it. is there a better way to do it or clean up the code?
@dataclass
class Price:
"""
This describes how to map default price value for product
"""
profit: float = 0.5
cost: float = 0.1
sale: float = 25.0
def round(self, n: float):
if n < 1:
return round(n, 2)
elif n < 100:
n = round(n / 1)
elif n < 1000:
n = round(n / 5) * 5
elif n < 10000:
n = round(n / 50) * 50
else:
n = round(n / 500) * 500
return n
def update(self, **kwargs):
rate = kwargs.get('rate', 1)
for k, v in asdict(self).items():
if k != 'sale':
v = self.round(v * rate)
v = kwargs.get(k) or v
setattr(self, k, float(v))
#run test
p = Price()
p.update(rate=1) #p = Price(profit=0.5, cost=0.1, sale=25.0)
p = Price()
p.update(**sale=450, **dict(rate=0.9073)) #p = Price(profit=0.45, cost=0.1, sale=450.0)
p = Price()
p.update(**sale=800, **dict(rate=301.377)) #p = Price(profit=150.0, cost=0.1, sale=800.0)
p = Price()
p.update(rate=301.377) #p = Price(profit=150.0, cost=0.1, sale=7550.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=1) #p = Price(profit=0.0, cost=0.5, sale=50.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=600, **dict(rate=0.9073)) #p = Price(profit=0.0, cost=0.5, sale=600.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=1200, **dict(rate=301.377)) #p = Price(profit=0.0, cost=0.5, sale=1200.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=301.377) #p = Price(profit=0.0, cost=0.5, sale=15000.0)
python python-3.x
$endgroup$
add a comment
|
$begingroup$
I have following code to create a mutable namedtuple. based my understand I can use dataclass to do it. is there a better way to do it or clean up the code?
@dataclass
class Price:
"""
This describes how to map default price value for product
"""
profit: float = 0.5
cost: float = 0.1
sale: float = 25.0
def round(self, n: float):
if n < 1:
return round(n, 2)
elif n < 100:
n = round(n / 1)
elif n < 1000:
n = round(n / 5) * 5
elif n < 10000:
n = round(n / 50) * 50
else:
n = round(n / 500) * 500
return n
def update(self, **kwargs):
rate = kwargs.get('rate', 1)
for k, v in asdict(self).items():
if k != 'sale':
v = self.round(v * rate)
v = kwargs.get(k) or v
setattr(self, k, float(v))
#run test
p = Price()
p.update(rate=1) #p = Price(profit=0.5, cost=0.1, sale=25.0)
p = Price()
p.update(**sale=450, **dict(rate=0.9073)) #p = Price(profit=0.45, cost=0.1, sale=450.0)
p = Price()
p.update(**sale=800, **dict(rate=301.377)) #p = Price(profit=150.0, cost=0.1, sale=800.0)
p = Price()
p.update(rate=301.377) #p = Price(profit=150.0, cost=0.1, sale=7550.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=1) #p = Price(profit=0.0, cost=0.5, sale=50.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=600, **dict(rate=0.9073)) #p = Price(profit=0.0, cost=0.5, sale=600.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=1200, **dict(rate=301.377)) #p = Price(profit=0.0, cost=0.5, sale=1200.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=301.377) #p = Price(profit=0.0, cost=0.5, sale=15000.0)
python python-3.x
$endgroup$
$begingroup$
Any particular reason you're not using python's built-inround
function orDecimal
class?
$endgroup$
– scnerd
7 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago
add a comment
|
$begingroup$
I have following code to create a mutable namedtuple. based my understand I can use dataclass to do it. is there a better way to do it or clean up the code?
@dataclass
class Price:
"""
This describes how to map default price value for product
"""
profit: float = 0.5
cost: float = 0.1
sale: float = 25.0
def round(self, n: float):
if n < 1:
return round(n, 2)
elif n < 100:
n = round(n / 1)
elif n < 1000:
n = round(n / 5) * 5
elif n < 10000:
n = round(n / 50) * 50
else:
n = round(n / 500) * 500
return n
def update(self, **kwargs):
rate = kwargs.get('rate', 1)
for k, v in asdict(self).items():
if k != 'sale':
v = self.round(v * rate)
v = kwargs.get(k) or v
setattr(self, k, float(v))
#run test
p = Price()
p.update(rate=1) #p = Price(profit=0.5, cost=0.1, sale=25.0)
p = Price()
p.update(**sale=450, **dict(rate=0.9073)) #p = Price(profit=0.45, cost=0.1, sale=450.0)
p = Price()
p.update(**sale=800, **dict(rate=301.377)) #p = Price(profit=150.0, cost=0.1, sale=800.0)
p = Price()
p.update(rate=301.377) #p = Price(profit=150.0, cost=0.1, sale=7550.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=1) #p = Price(profit=0.0, cost=0.5, sale=50.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=600, **dict(rate=0.9073)) #p = Price(profit=0.0, cost=0.5, sale=600.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=1200, **dict(rate=301.377)) #p = Price(profit=0.0, cost=0.5, sale=1200.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=301.377) #p = Price(profit=0.0, cost=0.5, sale=15000.0)
python python-3.x
$endgroup$
I have following code to create a mutable namedtuple. based my understand I can use dataclass to do it. is there a better way to do it or clean up the code?
@dataclass
class Price:
"""
This describes how to map default price value for product
"""
profit: float = 0.5
cost: float = 0.1
sale: float = 25.0
def round(self, n: float):
if n < 1:
return round(n, 2)
elif n < 100:
n = round(n / 1)
elif n < 1000:
n = round(n / 5) * 5
elif n < 10000:
n = round(n / 50) * 50
else:
n = round(n / 500) * 500
return n
def update(self, **kwargs):
rate = kwargs.get('rate', 1)
for k, v in asdict(self).items():
if k != 'sale':
v = self.round(v * rate)
v = kwargs.get(k) or v
setattr(self, k, float(v))
#run test
p = Price()
p.update(rate=1) #p = Price(profit=0.5, cost=0.1, sale=25.0)
p = Price()
p.update(**sale=450, **dict(rate=0.9073)) #p = Price(profit=0.45, cost=0.1, sale=450.0)
p = Price()
p.update(**sale=800, **dict(rate=301.377)) #p = Price(profit=150.0, cost=0.1, sale=800.0)
p = Price()
p.update(rate=301.377) #p = Price(profit=150.0, cost=0.1, sale=7550.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=1) #p = Price(profit=0.0, cost=0.5, sale=50.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=600, **dict(rate=0.9073)) #p = Price(profit=0.0, cost=0.5, sale=600.0)
p = Price(0.0, 0.5, 50.0)
p.update(**sale=1200, **dict(rate=301.377)) #p = Price(profit=0.0, cost=0.5, sale=1200.0)
p = Price(0.0, 0.5, 50.0)
p.update(rate=301.377) #p = Price(profit=0.0, cost=0.5, sale=15000.0)
python python-3.x
python python-3.x
edited 5 hours ago


200_success
136k21 gold badges175 silver badges445 bronze badges
136k21 gold badges175 silver badges445 bronze badges
asked 9 hours ago
jacobcan118jacobcan118
1866 bronze badges
1866 bronze badges
$begingroup$
Any particular reason you're not using python's built-inround
function orDecimal
class?
$endgroup$
– scnerd
7 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago
add a comment
|
$begingroup$
Any particular reason you're not using python's built-inround
function orDecimal
class?
$endgroup$
– scnerd
7 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago
$begingroup$
Any particular reason you're not using python's built-in
round
function or Decimal
class?$endgroup$
– scnerd
7 hours ago
$begingroup$
Any particular reason you're not using python's built-in
round
function or Decimal
class?$endgroup$
– scnerd
7 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago
add a comment
|
1 Answer
1
active
oldest
votes
$begingroup$
In short:
dataclass
is the right thing to use as a mutable named tuple. It's basically custom-built to be a great version of that idea.Use
decimal.Decimal
for any financial numbers.Don't re-implement
round
. There's a built-in version that behaves in nice, configurable ways with the Decimal object.Not sure what the
p.update(**sale=600, **dict(rate=0.9073))
is all about, just usep.update(sale=600, rate=0.9073)
(except with Decimals)
$endgroup$
$begingroup$
what should I use build-inround
version ? based on the logic of round I have?
$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use toround
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050
$endgroup$
– jacobcan118
4 hours ago
add a comment
|
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/4.0/"u003ecc by-sa 4.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f228917%2fmutable-named-tuple-with-default-value-and-conditional-rounding-support%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
In short:
dataclass
is the right thing to use as a mutable named tuple. It's basically custom-built to be a great version of that idea.Use
decimal.Decimal
for any financial numbers.Don't re-implement
round
. There's a built-in version that behaves in nice, configurable ways with the Decimal object.Not sure what the
p.update(**sale=600, **dict(rate=0.9073))
is all about, just usep.update(sale=600, rate=0.9073)
(except with Decimals)
$endgroup$
$begingroup$
what should I use build-inround
version ? based on the logic of round I have?
$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use toround
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050
$endgroup$
– jacobcan118
4 hours ago
add a comment
|
$begingroup$
In short:
dataclass
is the right thing to use as a mutable named tuple. It's basically custom-built to be a great version of that idea.Use
decimal.Decimal
for any financial numbers.Don't re-implement
round
. There's a built-in version that behaves in nice, configurable ways with the Decimal object.Not sure what the
p.update(**sale=600, **dict(rate=0.9073))
is all about, just usep.update(sale=600, rate=0.9073)
(except with Decimals)
$endgroup$
$begingroup$
what should I use build-inround
version ? based on the logic of round I have?
$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use toround
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050
$endgroup$
– jacobcan118
4 hours ago
add a comment
|
$begingroup$
In short:
dataclass
is the right thing to use as a mutable named tuple. It's basically custom-built to be a great version of that idea.Use
decimal.Decimal
for any financial numbers.Don't re-implement
round
. There's a built-in version that behaves in nice, configurable ways with the Decimal object.Not sure what the
p.update(**sale=600, **dict(rate=0.9073))
is all about, just usep.update(sale=600, rate=0.9073)
(except with Decimals)
$endgroup$
In short:
dataclass
is the right thing to use as a mutable named tuple. It's basically custom-built to be a great version of that idea.Use
decimal.Decimal
for any financial numbers.Don't re-implement
round
. There's a built-in version that behaves in nice, configurable ways with the Decimal object.Not sure what the
p.update(**sale=600, **dict(rate=0.9073))
is all about, just usep.update(sale=600, rate=0.9073)
(except with Decimals)
answered 7 hours ago
scnerdscnerd
1,3091 silver badge9 bronze badges
1,3091 silver badge9 bronze badges
$begingroup$
what should I use build-inround
version ? based on the logic of round I have?
$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use toround
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050
$endgroup$
– jacobcan118
4 hours ago
add a comment
|
$begingroup$
what should I use build-inround
version ? based on the logic of round I have?
$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use toround
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050
$endgroup$
– jacobcan118
4 hours ago
$begingroup$
what should I use build-in
round
version ? based on the logic of round I have?$endgroup$
– jacobcan118
7 hours ago
$begingroup$
what should I use build-in
round
version ? based on the logic of round I have?$endgroup$
– jacobcan118
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
Because the builtin version does all yours does, and is tested against all manner of edge cases that yours is not. And if it ever becomes important, it's also faster. So you can just remove the method.
$endgroup$
– Gloweye
7 hours ago
$begingroup$
so how can i use to
round
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050$endgroup$
– jacobcan118
4 hours ago
$begingroup$
so how can i use to
round
in the same logic? 102 -> round to 100, 103 -> round to 5 , 1023 -> 1000, 1044 -> 1050$endgroup$
– jacobcan118
4 hours ago
add a comment
|
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f228917%2fmutable-named-tuple-with-default-value-and-conditional-rounding-support%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qfeTnjlH93eRJRbng QhvS3,w0dFfjj s0llt nPclSnSW6rB9Y2IOhl,zlZe 4GWRWJaHlSl5fRjAvtgR,TZLfVwhS4UMkMO,s8YKeCn
$begingroup$
Any particular reason you're not using python's built-in
round
function orDecimal
class?$endgroup$
– scnerd
7 hours ago
$begingroup$
It's fairly clear what you mean by this question, although there are some terminology issues. Tuples by definition are immutable.
$endgroup$
– Reinderien
3 hours ago